Getting Started with Diffblue Cover
Diffblue Cover is an AI-powered software testing tool that generates unit tests for Java code. Tests can easily be generated directly from your code using the Diffblue Cover IntelliJ plugin. Let’s get started by setting up Diffblue Cover and start generating tests.
Intellij Plugin
Diffblue Cover provides an IntelliJ plugin that helps with interacting with Diffblue Cover.
The plugin displays the following icons next to your code so that you know what Diffblue Cover can provide to you in that context.
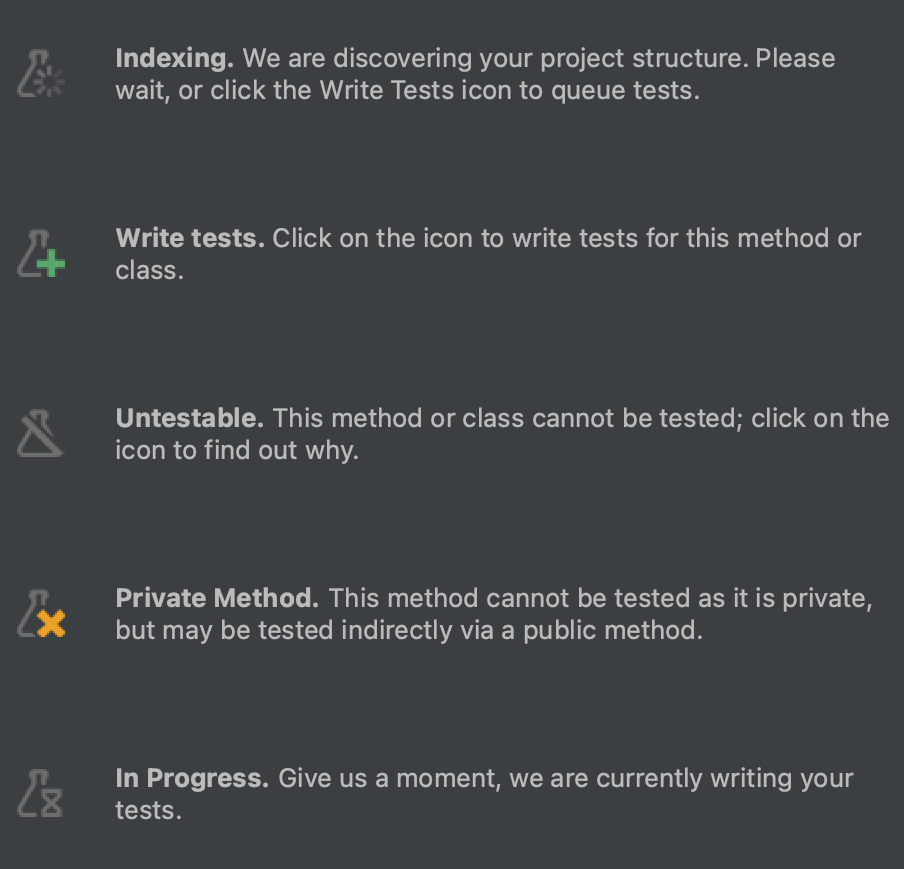
This is what the icons look like on your code. Based on the testability of your code Diffblue will display different icons.
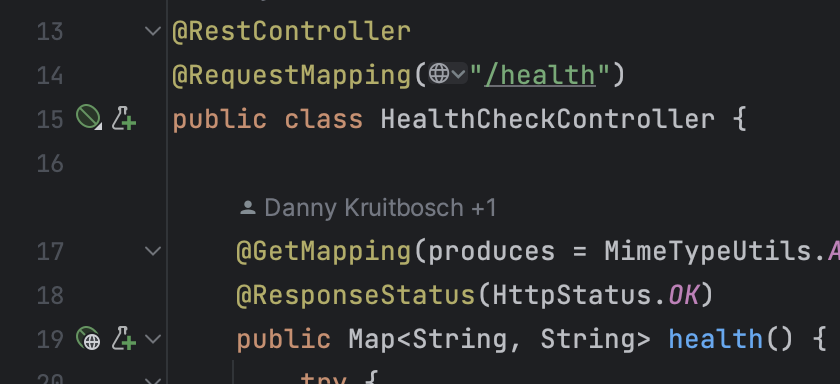
By clicking on the icon next to a method you can ask Diffblue Cover to write tests for you. Let’s start with some simple controller-level tests to see if everything is working.
Health Controller Test
Let’s have Diffblue Cover write some tests for a simple health controller. Below we have a controller that we would like to test
@RestController
@RequestMapping("/health")
public class HealthCheckController {
@GetMapping(produces = MimeTypeUtils.APPLICATION_JSON_VALUE)
@ResponseStatus(HttpStatus.OK)
public Map<String, String> health() {
try {
Map<String, String> resp = new HashMap<>();
resp.put("status", "OK");
return resp;
} catch (Exception ex) {
throw ExceptionTranslator.translate("HealthCheckController.health", ex);
}
}
}
After asking Diffblue Cover to create tests for us we have a short wait and then the following is displayed in IntelliJ.
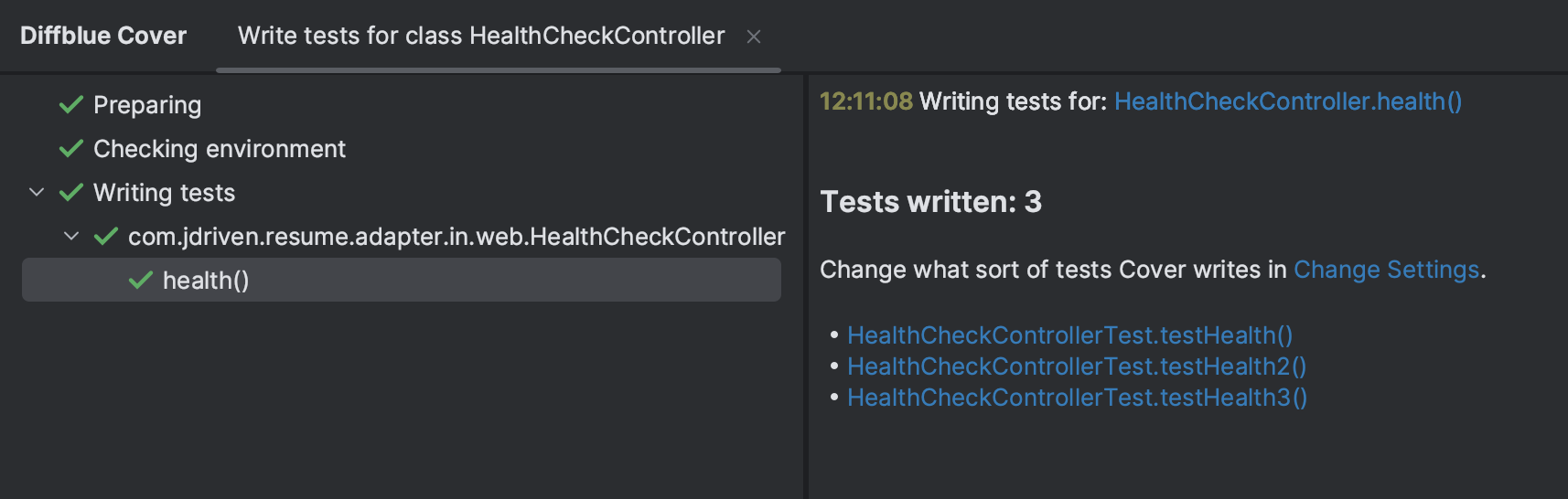
These tests were generated. No additional context was provided.
package com.jdriven.adapter.in.web;
import org.junit.Test;
import org.junit.runner.RunWith;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.security.test.web.servlet.request.SecurityMockMvcRequestBuilders;
import org.springframework.test.context.ContextConfiguration;
import org.springframework.test.context.junit4.SpringJUnit4ClassRunner;
import org.springframework.test.web.servlet.ResultActions;
import org.springframework.test.web.servlet.request.MockHttpServletRequestBuilder;
import org.springframework.test.web.servlet.request.MockMvcRequestBuilders;
import org.springframework.test.web.servlet.result.MockMvcResultMatchers;
import org.springframework.test.web.servlet.setup.MockMvcBuilders;
@ContextConfiguration(classes = {HealthCheckController.class})
@RunWith(SpringJUnit4ClassRunner.class)
public class HealthCheckControllerTest {
@Autowired
private HealthCheckController healthCheckController;
/**
* Method under test: {@link HealthCheckController#health()}
*/
@Test
public void testHealth() throws Exception {
MockHttpServletRequestBuilder requestBuilder = MockMvcRequestBuilders.get("/health");
MockMvcBuilders.standaloneSetup(healthCheckController)
.build()
.perform(requestBuilder)
.andExpect(MockMvcResultMatchers.status().isOk())
.andExpect(MockMvcResultMatchers.content().contentType("application/json"))
.andExpect(MockMvcResultMatchers.content().string("{\"status\":\"OK\"}"));
}
/**
* Method under test: {@link HealthCheckController#health()}
*/
@Test
public void testHealth2() throws Exception {
MockHttpServletRequestBuilder requestBuilder = MockMvcRequestBuilders.get("/health", "Uri Vars");
MockMvcBuilders.standaloneSetup(healthCheckController)
.build()
.perform(requestBuilder)
.andExpect(MockMvcResultMatchers.status().isOk())
.andExpect(MockMvcResultMatchers.content().contentType("application/json"))
.andExpect(MockMvcResultMatchers.content().string("{\"status\":\"OK\"}"));
}
/**
* Method under test: {@link HealthCheckController#health()}
*/
@Test
public void testHealth3() throws Exception {
SecurityMockMvcRequestBuilders.FormLoginRequestBuilder requestBuilder = SecurityMockMvcRequestBuilders
.formLogin();
ResultActions actualPerformResult = MockMvcBuilders.standaloneSetup(healthCheckController)
.build()
.perform(requestBuilder);
actualPerformResult.andExpect(MockMvcResultMatchers.status().isNotFound());
}
}
Conclusion
That was a simple example of how you can use Diffblue Cover to write tests for you. In a future article, we will cover using Diffblue Cover to test existing code.